Blob 表示的是二进制大文件,Binary Large Object.
Blob 对象表示一个不可变,原始数据的类文件对象。Blob 表示的不一定是 javascript 的原生格式的数据,File 接口基于 Blob,继承了 Blob 的功能并将其扩展使其支持用户系统上的文件。
要从其他非 blob 对象和数据构造一个 Blob,请使用 Blob() 构造函数。
1 2
| const blob = new Blob(); console.log(blob);
|
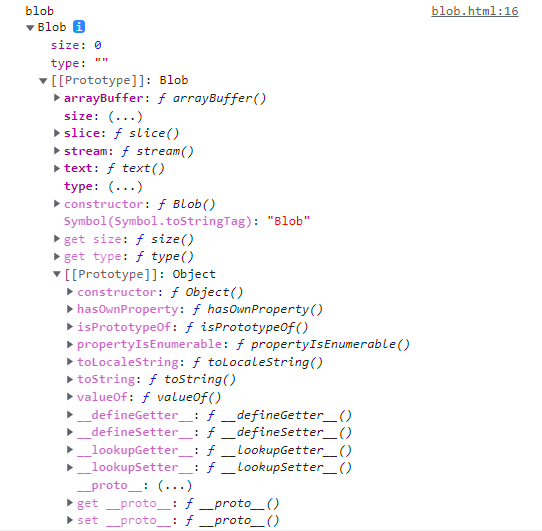
比如说
1 2 3 4 5 6 7 8 9 10 11 12
| const arr = [1, 2, 3];
const blob = new Blob([arr], { type: "text/plain", });
blob.text().then((res) => { console.log(res); });
|
指定为一个文件并下载
1 2
| <a href="" download id="dlbtn">下载</a>
|
1 2 3 4 5 6 7 8 9
| const html = `<h1>Nagisa</h1>`;
const nagisa = new Blob([html], { type: "text/html", }); dlbtn.onclick = function (e) { this.setAttribute("download", "nagisa.html"); this.href = URL.createObjectURL(nagisa); };
|
上传资源下载
1
| <input type="file" id="input" />
|
1 2 3 4 5 6 7 8 9 10
| input.onchange = function (e) { const files = e.target.files[0]; console.log("files", files);
const a = document.createElement("a"); a.setAttribute("download", "test.html"); const blob = new Blob([files], { type: "text/html" }); a.href = URL.createObjectURL(blob); a.click(); };
|
图片上传回显
1
| <input type="file" id="input" />
|
1 2 3 4 5 6 7
| input.onchange = function (e) { const file = e.target.files[0]; console.log(" ", file); const img = new Image(); img.src = URL.createObjectURL(file); document.body.appendChild(img); };
|